Software engineer interview questions are designed to assess technical skills, interpersonal competence, cultural fit, and more. HR professionals use these to assess job seekers for positions in their companies.
In this article, we’ll explore some of the questions you’re most likely to encounter, regardless of whether you’re a senior or a junior software engineer. We’ll explain the rationale behind them and provide examples of responses to show you how it should be done. Let’s get started!
Key Takeaways
Software engineer interview questions vary from general to highly technical and behavioral ones.
Technical questions assess your knowledge and experience in programming languages, algorithms, version control systems, specialized tools and techniques, and more.
Behavioral questions examine how you act in specific circumstances and are best answered using the structured STAR (Situation, Task, Action, Result) method.
To prepare for a software engineering interview, you should polish your technical knowledge and skills, research the company, and practice with a mock interview.
14 Technical Software Engineer Interview Questions & Sample Answers
Technical interview questions for software engineers evaluate your technical skills, coding knowledge, debugging proficiency, version control experience, etc. Let’s explore the standard ones that you’re most likely to encounter.
#1. What is your experience with programming languages?
Proficiency in programming languages is one of the core skills for software engineers. When recruiters ask this question, they want to see whether you’re experienced in languages used in their tech stack.
When responding to this coding interview question, you want to focus on the languages you’re most proficient in and provide some examples of your work. For bonus points, you can emphasize that you’re adept at quickly learning new languages.
Here’s an example of a good answer:
Good Example
“I have plenty of experience in JavaScript, PHP, and Python. I’ve used JavaScript and PHP for web development and Python for data processing. On top of that, I frequently use C++ for applications where performance is paramount. Additionally, I’m always open to learning new languages; for instance, I recently picked up Go for cross-platform desktop applications.”
#2. Can you explain the difference between object-oriented programming and functional programming?
This is a common interview question for entry-level software engineers designed to assess your understanding of fundamental programming concepts.
When answering the interview question about object-oriented design and functional programming, you want to explain the differences between the two and emphasize each one's strengths and weaknesses. This demonstrates that you have the flexibility to approach problems from different angles.
Let’s see an example:
Good Example
“Object-oriented programming leverages concepts like encapsulation, abstraction, and polymorphism to create objects with data and behavior built-in. It’s perfect for large-scale projects, as it allows optimal structuring.
On the other hand, functional programming is better suited for smaller projects that require simple inputs and outputs and pure calculations.”
#3. How do you approach debugging code?
Recruiters ask this software engineer interview question to examine your problem-solving skills and debugging methodology. Code debugging is an essential skill for software engineers, which is why you want to describe specific techniques and tools you use.
To make an even stronger impression, you can provide an example of how you handled a particularly tricky bug.
Here’s an example:
Good Example
“I approach code debugging by reproducing the problem to analyze and understand it better first. I check logs and error messages for information that could help me and, if needed, use breakpoints to step through the code.
For instance, in one of my recent projects, an API was returning incorrect data. I added logging statements to test the code with controlled inputs, discovering that the problem was related to an incorrect database query.”
#4. What is your experience with version control systems like Git?
This software engineer interview question gauges your proficiency in workflows that include version control and the ability to collaborate on projects.
Your response should demonstrate an in-depth understanding of branching strategies and experience in resolving conflicts. Moreover, you should highlight your ability to work with others using Git (or other version control systems).
Let’s see that in an example of a good answer:
Good Example
“I use Git for version control daily. Some of the commands I’m most comfortable with include ‘git rebase, ‘git cherry-pick,’ and ‘git bisect.’
In one of my recent projects, I helped resolve merge conflicts by analyzing diff files before collaborating with teammates to implement changes.”
#5. How do you ensure the scalability and performance of your code?
Interviewers ask this question to see whether you’re adept at writing efficient code that performs well and is easy to maintain.
When answering, you should emphasize your understanding of performance bottlenecks. Make sure to mention specific techniques you use to make your code scalable and efficient, such as profiling, caching, or parallel processing.
Here’s an example you can follow:
Good Example
“To ensure the scalability and performance of my code, I focus on minimizing expensive operations while using efficient data structures and algorithm optimization. For example, if I want to speed up queries, I’d leverage indexing, caching, and load balancing.
For instance, in one of my previous projects, I implemented query caching and request batching to reduce API response times by 61%.”
#6. What is your experience with cloud technologies (AWS, Azure, Google Cloud)?
Recruiters ask this cloud computing interview question to assess your proficiency in relevant tools and techniques. They want to see if you’re comfortable working with cloud-based infrastructure, including experience in deployment and security aspects.
A good answer should include the specific cloud platforms and services you used, including an example of how you applied those skills in a project.
Here’s a good example:
Good Example
“I have extensive experience working with AWS. I’ve used it to host applications, manage databases, and set up serverless functions. In my most recent project, I used AWS Lambda in combination with S3 for task processing and storage, reducing the company’s server costs.
Additionally, I have set up multiple virtual machines on Azure and I deployed five applications on Google Cloud’s App Engine.”
#7. Can you explain the concept of multithreading?
When recruiters ask this software engineer interview question, they want to see whether you can write code that runs efficiently on modern processors.
Here, you want to demonstrate an understanding of concurrency and show how you handle specific issues, such as race conditions and deadlocks.
A good answer looks like this:
Good Example
“Multithreading allows programs to run multiple tasks simultaneously, often significantly improving their performance. This is usually done with tasks that aren’t dependent on each other, cutting the time it takes to perform them.
For instance, if I’m making a chat application, I can leverage multithreading to have one thread handle user input while the other processes messages in the background. I’ve frequently used multithreading in data processing to reduce the time it takes to handle large volumes of data by up to 50%.”
#8. What is the importance of software testing, and what types of tests do you use?
Software testing is crucial to making programs stable and reliable. When recruiters ask this software developer interview question, they are interested in seeing how you ensure code quality, which types of tests you’re familiar with, and what your experience is in manual and automated testing.
Your response should explain the importance of testing and mention your experience with different types of tests.
Let’s see that in an example of a good answer:
Good Example
“Software testing is essential in catching bugs early in development, preventing code regressions, and ensuring overall reliability. I use unit tests to examine individual functions, while integration tests help me verify if different parts of the project work together.
I also regularly use end-to-end tests to check entire applications. In my last role, we had company-wide automation tests to run before every deployment to save a lot of time on manual testing.”
#9. Can you explain the concept of APIs and how you’ve worked with them?
This software engineer interview question is designed to assess your understanding of APIs and how they connect different systems.
When answering, you want to talk about your experiences creating APIs or integrating them into applications. You should also mention different details about specific types of APIs, like REST or GraphQL.
Here’s an example of an answer to this interview question:
Good Example
“An API stands for ‘Application Programming Interface’ and enables communication between different systems. For instance, when building a weather application, you can integrate a temperature API that would fetch temperature data from another server.
Most of my experience with APIs revolves around building REST APIs to receive and send data in JSON format. For instance, I built an API that retrieves product details for an e-commerce client and integrated third-party payment APIs, like Stripe.”
#10. What tools do you use for continuous integration/continuous deployment (CI/CD)?
Continuous integration and deployment are essential for development efficiency. When recruiters ask this question, they want to see your experience with CI/CD pipelines, whether you’re familiar with DevOps practices, and how you approach automated testing and deployment.
A good response should highlight tools and techniques used to improve the workflow.
Let’s see that in an example:
Good Example
“Some of the CI/CD tools I am familiar with and have used in the past include Jenkins, GitHub Actions, and GitLab CI. In my previous role, we set up a pipeline to automatically test every change in code and deploy updates to a staging server. This approach significantly reduced manual effort and improved our efficiency.”
#11. How do you approach writing efficient algorithms?
Algorithm interview questions simultaneously analyze your coding experience and problem-solving skills.
Your answer to this question should demonstrate a thoughtful approach to coding where you continuously think about performance and optimization while understanding time and space complexity.
Here’s an example of what a good answer should look like:
Good Example
“In my approach to writing efficient algorithms, I focus on minimizing unnecessary computations and choosing the right data structures. When I work with large datasets, I use scalable sorting and searching algorithms.
For example, I worked on a feature that involved going through thousands of records, initially designed to use linear search. This approach was too slow, so I switched to a hash map and reduced lookup times to milliseconds.”
#12. What is your experience with front-end technologies (HTML, CSS, JavaScript)?
This is a common interview question for a front-end developer. Its goal is to gauge the applicant’s experience in building user interfaces, as well as their knowledge of UI/UX principles.
One of the best ways to answer this question is by discussing a specific project you worked on.
Let’s see that in an example:
Good Example
“I have experience in HTML, CSS, and JavaScript, including React and Vue frameworks. During my previous employment, I built a dynamic dashboard for a finance client, which displayed real-time data through engaging graphics. By making a design responsive, I made it accessible for different screen sizes and devices.”
#13. What is your experience with databases (SQL/NoSQL)?
Recruiters ask this software engineer interview question to assess your ability to work with data, whether by storing, managing, or retrieving it.
When giving a response, you should showcase an understanding of relational and non-relational databases and demonstrate the ability to design and optimize queries. If you have any experience working with cloud-based databases, you should mention that to strengthen your case.
Here’s an example:
Good Example
“I have experience with PostgreSQL and MySQL for structured data and MongoDB as a NoSQL database for flexible storage. For example, in one of my projects for a fitness app, I used PostgreSQL to store user profiles and MongoDB for real-time chat messages. Indexing helped me optimize to improve performance.”
#14. How do you ensure the security of your code?
This software engineer interview question probes into your knowledge of common security threats. Recruiters ask this to see your familiarity with practices like authentication and encryption, but they also want to see how you prevent vulnerabilities.
An optimal answer should showcase theoretical knowledge combined with an example of a situation when you applied your skills.
Let’s see what that might look like:
Good Example
“To ensure the security of my code, I follow the best practices, including input validation, encryption, and authentication methods. In one of my recent projects, I kept the user data safe by implementing JWT-based authentication, while parameterized queries helped prevent SQL injections.”
5 Behavioral Software Engineer Interview Questions & Sample Answers

Behavioral interview questions for software engineers help recruiters examine how you performed in specified situations, helping them predict how you might perform in the future.
When responding to these questions, you should use the STAR method (Situation, Task, Action, Result) to provide a concise, well-structured, and informational answer.
#1. Can you describe a challenging project you've worked on and how you handled it?
This is one of the basic problem-solving questions for software engineers. Recruiters ask this to see how you tackle problems and handle setbacks, whether you’re good at collaborating with others, and if you can learn from failures and improve yourself.
One of the best ways to answer the question is by picking a specific project where you overcame a challenge.
The example may look like this:
Sample Answer
“I worked on a project where we had to migrate a large application under a tight deadline, yet some libraries weren’t compatible with the new framework.
I collaborated with the team to rewrite parts of the code while researching for an alternative solution. We implemented custom adapters where needed, completed migration on time, and improved application performance.”
#2. How do you stay updated with the latest software development trends?
The purpose of this software engineer interview question is to examine your willingness to learn and stay current in your field. Employers always look for candidates who keep up with new technologies and apply newfound knowledge in their work. They become more efficient over time and quickly adapt to changing circumstances.
When responding, you should mention methods and resources you use, such as courses, blogs (e.g., Dev.to), conferences, etc.
Here’s a good way to answer this question:
Sample Answer
“To stay updated on the latest trends and developments, I follow several tech blogs on Medium and Dev.to. On top of that, I regularly attend conferences and watch online talks. Plus, I participate in discussions on Stack Overflow and GitHub.
I recently took an online course on Svelte and built a side project to experiment with it and understand its advantages over more established frameworks.”
#3. How would you handle tight deadlines and prioritize tasks?
This question probes into your prioritization and time management skills, as well as your ability to work well under pressure. Here, you want to demonstrate the ability to handle stress, manage your workload, and communicate with the team and stakeholders to ensure deadline alignment.
A good answer should look like this:
Sample Answer
“When I have to handle tight deadlines, I split my workload into small tasks to make them more manageable. I use the prioritization matrix to determine critical tasks and finish the first while leveraging project management tools to track overall progress and ensure I don’t miss anything important.
I also aim to have transparent communication with the team and stakeholders to ensure everyone is on the same page until we deliver the project.”
#4. Have you worked with Agile or Scrum methodologies?
Agile and Scrum are modern project management methodologies often utilized in software development. This is supposed to show whether you understand the core principles behind these methodologies and have some experience with them.
The best way to answer this question would be by discussing how you collaborated with a team on a project while practicing Agile or Scrum.
Here’s an example:
Sample Answer
“I was part of an Agile team practicing Scrum when developing a mobile app. We conducted daily stand-ups and had two-week sprints followed by retrospectives.
My main approach was breaking down tasks into more manageable user stories while collaborating with the product owner. By analyzing and optimizing requirements, we continuously improved development and quickly adapted to any changes.”
#5. Have you worked in a team with other engineers? How did you collaborate?
This software engineer interview question directly examines your communication and collaboration skills, as well as your ability to work in interdisciplinary teams.
Your response should show your ability to foster a positive team environment while also showcasing relevant soft skills.
It should look like this:
Sample Answer
“I’ve worked with multiple interdisciplinary teams where we used pair programming, code reviews, and frequent check-ins. I collaborated with one colleague using Git, and we regularly reviewed the other person’s code to provide feedback. This helped us maintain high quality while learning from each other.”
15 Additional Software Engineer Interview Questions
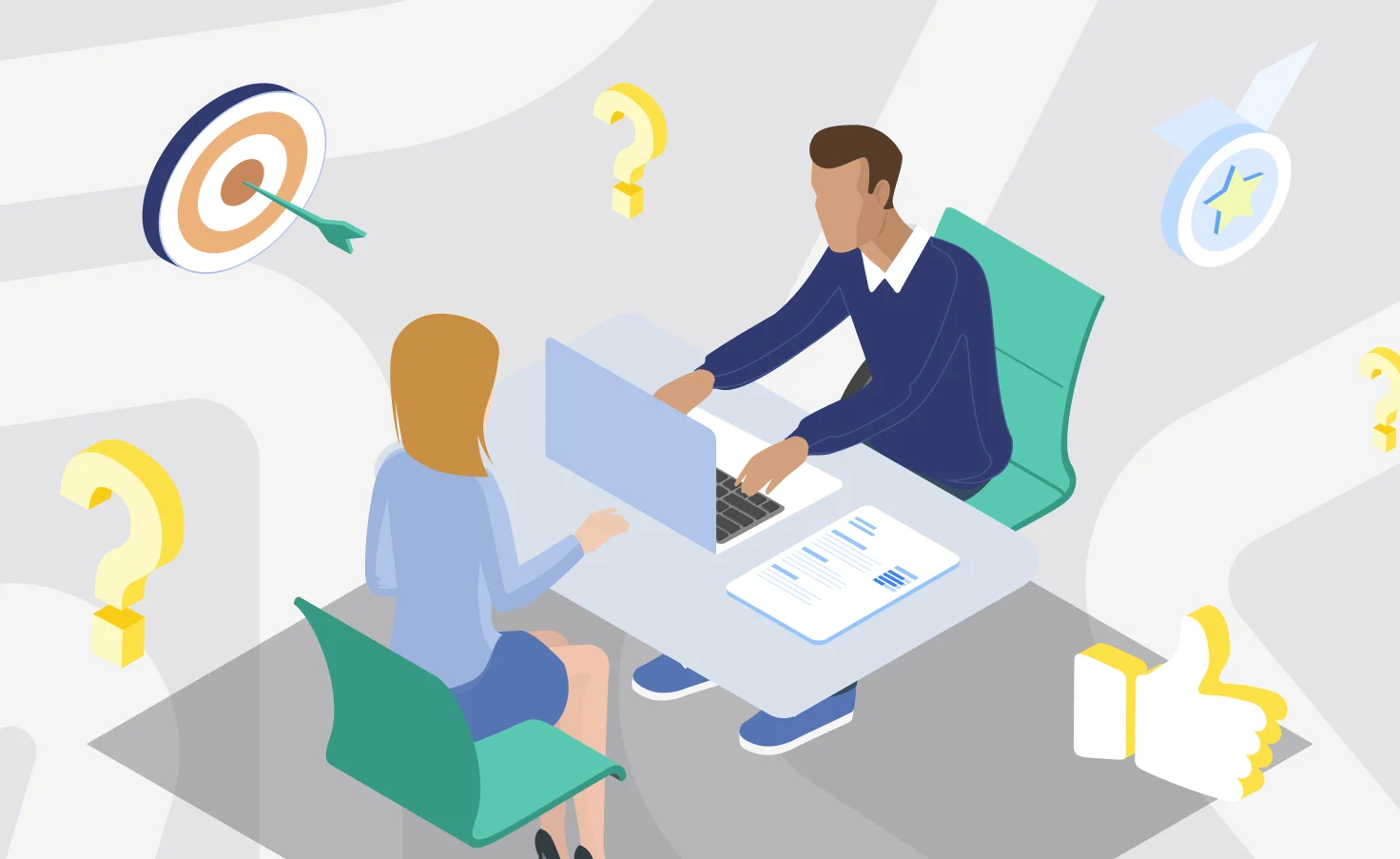
There are many additional software engineer interview questions recruiters can ask you. Let’s start with five general ones mostly used to assess candidates broadly:
General Interview Questions
Why are you leaving your current employer?
What are your greatest accomplishments?
Now, here are some system-design interview questions:
System-Design Interview Questions
How would you design a URL-shortening service?
How would you develop a real-time chat application?
What would you consider when creating a distributed database?
How would you handle peak-hour traffic on an e-commerce website?
How would you design a monitoring system for a cloud-based application?
Finally, here are five questions you might encounter if you’re applying for a software engineer role at Microsoft, FAANG, or another industry giant:
Top Software Engineer Interview Questions
Given a list of numbers, find the longest increasing subsequence.
Implement an LRU cache.
How would you design a global file storage system like Google Drive?
How would you scale a database for millions of users?
What strategies would you use to handle high read or write requests on a social media platform?
3 Foolproof Tips for Nailing Your Interview as a Software Engineer
Before we conclude the article, here are three expert tips that will elevate your software engineering interview preparation to the next level:
Software Engineer Interview Tips
Master your technical skills. Many tech companies will thoroughly test your theoretical knowledge, problem-solving skills, and coding aptitude. To prepare, you can practice coding on websites like LeetCode, polish your understanding of arrays, linked lists, or dynamic programming, design personal projects, apps, and systems, and more.
Research the company. By researching the company you’re applying to, you’ll find valuable information about its team, work, and culture. You can discover these details on the company’s website, social media, news articles, etc. This will help you figure out what they want in candidates and what type of questions you’re most likely to encounter.
Practice with a mock interview. Conducting a mock interview is one of the best ways to prepare for the meeting with the recruiter. You can ask a friend or a mentor to help you or even film yourself with a camera; this will help you find areas where you can improve, ensuring you impress the interviewer with knowledge and confidence.
Final Thoughts
Familiarizing yourself with the most common software engineer interview questions and answers is one of the best ways to prepare for a meeting with the recruiter who you just impressed with your resume. However, don’t forget to also polish your technical skills and practice giving answers in advance.
That way, you’ll significantly reduce any potential interview anxiety while communicating in a clear and confident manner. Keep these tips in mind whether you’re applying for a position in FAANG or an upcoming startup, and you’ll get the role you’re after sooner rather than later. Best of luck!